Advanced Techniques in Core Java Data Science Mobile Development Robust Frameworks Security
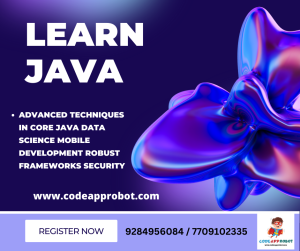
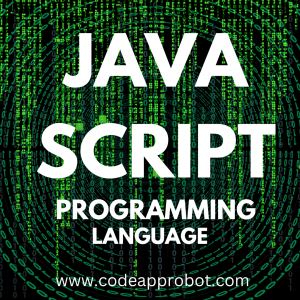
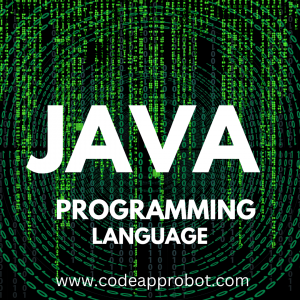
Advanced Techniques in Core Java Data Science Mobile Development Robust Frameworks Security
Advanced Techniques in Core Java Data Science Mobile Development Robust Frameworks Security
Java is a widely used programming language that is popular in both academia and industry. It is an object-oriented, class-based language that is designed to be portable, meaning that it can run on any platform that has a Java Virtual Machine (JVM) installed. Java is used to develop a wide variety of software applications, including web applications, mobile apps, desktop applications, and more.
The following is a detailed description of the syllabus for Java programming, including both core Java and advanced Java topics.
Core Java Syllabus:
- Introduction to Java
- History of Java
- Features of Java
- Advantages of Java
- Object-Oriented Programming
- Basic concepts of OOP
- Classes and objects
- Inheritance and polymorphism
- Abstract classes and interfaces
- Java Data Types
- Primitive data types
- Wrapper classes
- Arrays and strings
- Control Structures
- Conditional statements
- Loops
- Switch statements
- Exception Handling
- Try-catch blocks
- Multiple catch blocks
- Throws and throw statements
- Input and Output Streams
- File handling in Java
- Input and output streams
- Serialization and deserialization
- Collections Framework
- Introduction to the collections framework
- List, Set, and Map interfaces
- ArrayList, LinkedList, HashSet, and HashMap
- Multi-threading
- Introduction to threads
- Synchronization
- Deadlocks
- JDBC
- Introduction to JDBC
- Database connectivity
- SQL queries and statements
Advanced Java Syllabus:
- Servlets
- Introduction to Servlets
- Servlet life cycle
- Servlet configuration and initialization
- Java Server Pages (JSP)
- Introduction to JSP
- JSP life cycle
- JSP tags and directives
- Java Beans
- Introduction to Java Beans
- Properties, events, and methods
- Bean persistence
- Enterprise JavaBeans (EJB)
- Introduction to EJB
- Session, entity, and message-driven beans
- Container-managed persistence
- Spring Framework
- Introduction to Spring
- Spring modules
- Spring MVC architecture
- Hibernate Framework
- Introduction to Hibernate
- Hibernate architecture
- Hibernate mappings
- Web Services
- Introduction to web services
- SOAP and RESTful web services
- Creating and consuming web services
- Struts Framework
- Introduction to Struts
- Struts architecture
- Struts actions and forms
- Design Patterns
- Introduction to design patterns
- Creational, structural, and behavioral patterns
Overall, Java programming is a vast field with a wide range of topics to cover. By mastering both core and advanced Java topics, one can become a proficient Java developer capable of developing complex software applications.
Advanced Techniques in Core Java Data Science Mobile Development Robust Frameworks Security
Core Java Syllabus:
- Introduction to Java: Introduction to Java, History of Java, Features of Java, and Java Virtual Machine.
- Basics of Java Programming: Data types, Variables, Operators, and Expressions, Decision-making, and Looping.
- Classes and Objects: Classes and Objects, Constructors, Method Overloading, Encapsulation, and Inheritance.
- Packages and Interfaces: Packages, Interfaces, Access Specifiers, and Exception Handling.
- Multithreading: Multithreading, Synchronization, and Deadlocks.
- I/O Streams and Files: I/O Streams, Byte Streams, and Character Streams, and Files.
- Collections Framework: Introduction to Collections, ArrayList, LinkedList, Vector, and Iterator.
- JDBC: Introduction to JDBC, JDBC Architecture, and Steps to Connect to Database.
- Networking: Socket Programming, InetAddress, and URL Processing.
Advanced Java Syllabus:
- Servlets: Introduction to Servlets, Servlet Life Cycle, and Servlet API.
- JavaServer Pages (JSP): Introduction to JSP, Scripting Elements, Directive Elements, and Action Elements.
- Enterprise JavaBeans (EJB): Introduction to EJB, Types of EJB, Session Beans, Entity Beans, and Message-Driven Beans.
- Hibernate: Introduction to Hibernate, Hibernate Architecture, and Hibernate Configuration.
- Spring: Introduction to Spring, Spring Modules, Spring Architecture, and Spring Configuration.
- Struts: Introduction to Struts, Struts Architecture, and Struts Configuration.
- Java Messaging Service (JMS): Introduction to JMS, JMS Architecture, and JMS API.
- Web Services: Introduction to Web Services, SOAP, REST, and Web Services API.
More Details:
Advanced Techniques in Core Java:
- Data Science: Introduction to Data Science, Machine Learning, Python Integration, Apache Spark, and Hadoop.
- Mobile Development: Introduction to Mobile Development, Android Development, and iOS Development.
- Robust Frameworks: Introduction to Frameworks, Spring Framework, and Hibernate Framework.
- Security: Introduction to Security, Java Security, and Security Libraries.
Data Science:
- Data Analysis and Visualization: Data Analysis and Visualization Techniques, Data Science Tools and Libraries, and Data Preprocessing.
- Machine Learning: Introduction to Machine Learning, Supervised Learning, Unsupervised Learning, and Reinforcement Learning.
- Deep Learning: Introduction to Deep Learning, Neural Networks, Convolutional Neural Networks, and Recurrent Neural Networks.
- Natural Language Processing (NLP): Introduction to NLP, NLP Applications, NLP Libraries, and NLP Techniques.
Mobile Development:
- Android Development: Introduction to Android Development, Android Studio, Android Layouts, and Android Views.
- iOS Development: Introduction to iOS Development, Xcode, iOS Layouts, and iOS Views.
Robust Frameworks:
- Spring Framework: Introduction to Spring Framework, Spring Core, Spring Boot, and Spring Web MVC.
- Hibernate Framework: Introduction to Hibernate Framework, Hibernate ORM, Hibernate Annotations, and Hibernate Query Language.
Security:
- Java Security: Introduction to Java Security, Security APIs, and Security Frameworks.
- Security Libraries: Introduction to Security Libraries, Bouncy Castle, and Jasypt.
This syllabus covers a wide range of topics and techniques in Core Java, Advanced Java, Data Science, Mobile Development, Robust Frameworks, and Security. By mastering these topics, you will be equipped with the skills and knowledge to develop robust, secure, and scalable applications using Java.
Advanced Techniques in Core Java Data Science Mobile Development Robust Frameworks Security
JavaScript is a popular programming language used for front-end web development. It is a versatile language that allows for the creation of interactive web pages and web applications. In this article, we will discuss the fundamentals of JavaScript, its syntax, and the most commonly used programming features. We will also explore how JavaScript can be used to create dynamic user interfaces, manipulate HTML and CSS, and interact with server-side technologies.
Introduction to JavaScript: JavaScript is a high-level, interpreted programming language that is used to add interactivity and dynamic functionality to web pages. It was developed in 1995 by Brendan Eich, and has since become one of the most widely used programming languages in the world. It is primarily used for front-end web development, but can also be used on the server-side with Node.js.
Syntax: The syntax of JavaScript is similar to other programming languages such as Java and C++. JavaScript code is executed in a web browser, and is typically embedded within HTML code. JavaScript code is enclosed within script tags, and can be placed in the head or body section of an HTML document.
Variables: JavaScript uses the var keyword to declare variables. Variables can store numbers, strings, boolean values, or objects. Variables can also be used to store arrays, which are a collection of values. JavaScript is a dynamically-typed language, which means that the data type of a variable can change at runtime.
Functions: Functions are a fundamental feature of JavaScript. They are blocks of code that can be executed multiple times with different input parameters. Functions can be declared using the function keyword, and can accept parameters and return values.
Control Structures: JavaScript provides a variety of control structures that allow for conditional execution and looping. The if statement is used to execute code based on a specified condition. The switch statement is used to execute code based on a specified value. The for loop and while loop are used to execute code multiple times.
Events: JavaScript can be used to handle events that occur in a web page, such as button clicks, mouse movements, and keyboard inputs. Event handling in JavaScript involves creating event listeners that are triggered when an event occurs.
DOM Manipulation: The Document Object Model (DOM) is a programming interface for HTML and XML documents. JavaScript can be used to manipulate the DOM, allowing for the creation of dynamic web pages. DOM manipulation involves selecting HTML elements and changing their attributes and properties.
jQuery: jQuery is a popular JavaScript library that simplifies DOM manipulation and event handling. It provides a simple and concise API for interacting with HTML and CSS.
Asynchronous JavaScript: Asynchronous JavaScript allows for the execution of code without blocking the main thread. It is commonly used for making network requests, handling user input, and running background tasks. Promises and async/await are two popular features of JavaScript that are used for asynchronous programming.
Syllabus for Core Java:
- Introduction to Java
- Data types, Operators, and Expressions
- Control Structures
- Arrays and Strings
- Object-Oriented Programming Concepts
- Classes and Objects
- Inheritance and Polymorphism
- Exception Handling
- File Input/Output
- Graphical User Interfaces (GUI)
- Event Handling
- Multithreading
- Networking
Syllabus for Advanced Java:
- JDBC (Java Database Connectivity)
- Servlets and JSP (JavaServer Pages)
- JavaBeans
- RMI (Remote Method Invocation)
- EJB (Enterprise JavaBeans)
- Struts Framework
- Spring Framework
- Hibernate ORM (Object-Relational Mapping)
- Web Services
- JMS (Java Message Service)
- JMX (Java Management Extensions)
- Security
In conclusion, JavaScript is a powerful programming
Advanced Techniques in Core Java Data Science Mobile Development Robust Frameworks Security
some examples and resources to get started:
- Java Code Examples:
- Java Examples – Examples on Java programming concepts and constructs.
- Java Programming Examples – GeeksforGeeks – Examples of Java programs from basic to advanced concepts.
- Java Code Examples – Programiz – A collection of Java programming examples that demonstrate various features and functions.
- Advanced Java Code Examples:
- Advanced Java Programming Examples – Examples of advanced Java programming concepts like JDBC, Servlets, JSP, RMI, etc.
- Java EE 6 Samples – Oracle – A collection of samples for advanced Java programming using Java EE 6 technologies like EJB, JPA, JMS, etc.
- Advanced Java Programs – JournalDev – Examples of advanced Java programs like Multithreading, Collections, Annotations, etc.
- Core Java Code Examples:
- Core Java Examples – Examples of Core Java programming concepts like OOP, Exception Handling, File I/O, etc.
- Core Java Programs – Sanfoundry – A collection of Core Java programming examples and solutions.
- Core Java Projects – Project Topics – Examples of Core Java projects to practice programming concepts.
- JavaScript Code Examples:
- JavaScript Examples – Examples of JavaScript programming concepts like loops, arrays, functions, etc.
- JavaScript Projects – FreeCodeCamp – A collection of JavaScript projects for beginners to advanced developers.
- JavaScript Code Examples – Programiz – A collection of JavaScript programming examples that demonstrate various features and functions.
You can also find various tutorials, videos, and courses online to learn more about coding in Java, Advanced Java, Core Java, and JavaScript.
here are some frequently asked questions about Java programming, advanced Java, core Java, and JavaScript:
Java Programming:
- What is Java and why is it important?
- What are the key features of Java?
- How is Java different from other programming languages?
- What are the basic data types in Java?
- How do you write and run a Java program?
Advanced Java:
- What is advanced Java and how is it different from core Java?
- What are some popular frameworks used in advanced Java development?
- What are the key features of Spring Framework?
- How is Hibernate used in advanced Java development?
- What are the benefits of using JavaServer Faces (JSF) in web development?
Core Java:
- What is core Java and what are its basic concepts?
- What are the key features of Java 8?
- How do you handle exceptions in Java?
- What is multi-threading in Java and how is it implemented?
- What are the different types of classes in Java?
JavaScript:
- What is JavaScript and why is it important?
- How is JavaScript different from Java?
- What are some popular JavaScript libraries and frameworks?
- How do you use JavaScript to manipulate the Document Object Model (DOM)?
- What is asynchronous programming in JavaScript?