Advanced C++ Coding Techniques Mastering Data Structures Algorithms
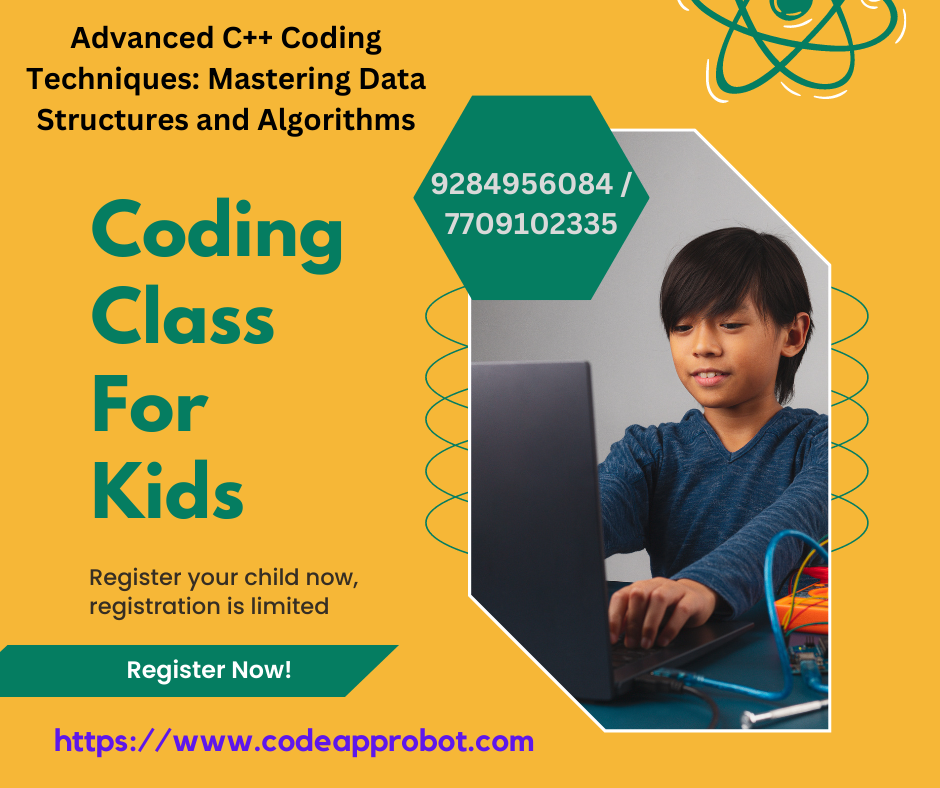
Advanced C++ Coding Techniques Mastering Data Structures Algorithms
Advanced C++ Coding Techniques Mastering Data Structures Algorithms
Introduction
C++ is a powerful and versatile programming language that is widely used in a variety of applications, from operating systems and device drivers to video games and finance. As a result, it is essential for developers to have a solid understanding of advanced C++ coding techniques and data structures.
In this article, we will explore some of the key concepts and techniques that are essential for mastering C++ data structures and algorithms. We will cover topics such as templates, iterators, smart pointers, and memory management, and demonstrate how these techniques can be used to improve the performance and efficiency of your C++ code.
Part 1: Templates
Templates are a powerful feature of C++ that enable developers to create generic code that can work with a wide variety of data types. In essence, templates allow you to define a “template” for a class or function that can be instantiated with different data types at runtime.
The most common use of templates is for creating container classes, such as vectors and lists, that can hold elements of any type. For example, the following code shows how to define a simple template for a container class:
template <typename T>
class Container {
public:
void add(const T& item) { m_items.push_back(item); }
T get(int index) { return m_items[index]; }
private:
vector<T> m_items;
};
This template defines a simple container class that can hold items of any type. The typename
keyword is used to specify the type of the template parameter, and the vector
class is used to implement the container.
Templates can also be used for creating generic algorithms that work with any type of data. For example, the following code shows how to define a template for a generic “max” function:
template <typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
This template defines a simple “max” function that can compare two values of any type. The typename
keyword is used to specify the type of the template parameter, and the function returns the larger of the two values.
Part 2: Iterators
Iterators are another powerful feature of C++ that enable developers to iterate over collections of data. In essence, iterators are objects that allow you to access and manipulate the elements of a container class in a flexible and efficient way.
There are two main types of iterators in C++: forward iterators and random access iterators. Forward iterators are used for iterating over a collection of data one element at a time, while random access iterators are used for accessing the elements of a collection in a random order.
The most common use of iterators is for iterating over the elements of a container class, such as a vector or a list. For example, the following code shows how to use a forward iterator to iterate over the elements of a vector:
vector<int> v = { 1, 2, 3, 4, 5 };
for (auto it = v.begin(); it != v.end(); ++it) {
cout << *it << endl;
}
This code uses the begin()
and end()
member functions of the vector
class to create a forward iterator that can be used to iterate over the elements of the vector. The *it
notation is used to access the value of each element in the vector.
Random access iterators are used for accessing the elements of a collection in a random order. For example, the following code shows how to use a random access iterator to access the elements of a vector:
vector<int> v = { 1, 2, 3, 4, 5 };
cout << “Element 3 = “
Advanced C++ Coding Techniques Mastering Data Structures Algorithms
Smart pointers: In addition to raw pointers, C++ also provides a range of smart pointers that manage the lifetime of dynamically allocated objects automatically. Some popular types of smart pointers include unique_ptr, shared_ptr, and weak_ptr. You can cover the differences between these types, their use cases, and how to implement them in your code.
Templates: C++ templates are a powerful tool for generic programming that allow you to write code that works with different data types. You can discuss how to create class templates and function templates, and how to specialize them for specific types or requirements.
STL Containers: The C++ Standard Template Library (STL) provides a set of container classes that can store and manage collections of objects. You can discuss some of the most commonly used STL containers, such as vector, list, map, and set, and how to use them in your code.
STL Algorithms: Along with containers, the STL also provides a range of algorithms that can be used to manipulate collections of data. Some of the most commonly used algorithms include sort, find, binary_search, and transform. You can discuss how to use these algorithms and how they can help improve the performance of your code.
Recursion: Recursion is a programming technique where a function calls itself to solve a problem. You can cover the basics of recursion, including how to write recursive functions and how to use recursion to solve complex problems.
Graph Algorithms: Graphs are a mathematical concept that can be used to model complex systems such as social networks, transportation systems, and computer networks. You can discuss some common graph algorithms, such as breadth-first search, depth-first search, Dijkstra’s algorithm, and the Bellman-Ford algorithm, and how to implement them in C++.
Divide and Conquer Algorithms: Divide and conquer is a technique for solving complex problems by breaking them down into smaller, simpler subproblems. You can discuss some common divide and conquer algorithms, such as merge sort, quicksort, and binary search, and how to implement them in C++.
Dynamic Programming: Dynamic programming is a technique for solving complex problems by breaking them down into smaller subproblems and storing the solutions to those subproblems in memory. You can cover some common dynamic programming algorithms, such as the Fibonacci sequence and the Knapsack problem, and how to implement them in C++.
Big O Notation: Big O notation is a way to describe the performance of an algorithm in terms of the size of its input. You can discuss how to calculate the time and space complexity of an algorithm, and how to use Big O notation to analyze and optimize your code.
Memory Management: Efficient memory management is crucial for writing high-performance C++ code. You can discuss some best practices for managing memory in C++, including avoiding memory leaks, using RAII (Resource Acquisition Is Initialization), and implementing custom memory allocators.
Advanced C++ Coding Techniques Mastering Data Structures Algorithms
Introduction to C++ Programming
Course Description: This course is an introduction to the C++ programming language. The course covers the basic syntax and semantics of the language, as well as object-oriented programming principles. The course also covers common data structures and algorithms, and students will develop skills in problem-solving and critical thinking.
Course Outline:
I. Introduction to C++ A. Overview of C++ B. Getting Started with C++ C. Variables and Data Types D. Operators and Expressions
II. Control Structures A. Conditional Statements B. Loops C. Functions D. Recursion
III. Object-Oriented Programming A. Classes and Objects B. Encapsulation and Data Hiding C. Inheritance and Polymorphism D. Virtual Functions
IV. Data Structures A. Arrays B. Pointers C. Dynamic Memory Allocation D. Linked Lists E. Stacks and Queues F. Trees
V. Algorithms A. Searching and Sorting Algorithms B. Recursion C. Dynamic Programming D. Greedy Algorithms
VI. File Input and Output A. Stream Classes B. File Handling
VII. Exceptions A. Exception Handling B. Error Handling
VIII. Advanced C++ Topics A. Templates B. Standard Template Library (STL) C. Multi-threading D. Graphics and GUI Programming
Assessment and Grading:
- Homework Assignments: 30%
- Programming Assignments: 40%
- Midterm Exam: 15%
- Final Exam: 15%
Advanced C++ Coding Techniques Mastering Data Structures Algorithms
Object-Oriented Programming (OOP): C++ is an OOP language which means that it is based on the concept of objects. An object is a software entity that stores data and provides functionality that can be used by other software entities. OOP provides a way to encapsulate data and functionality and makes it easier to manage and maintain code.
Templates: C++ supports templates, which are a way to write generic code that can be used with different data types. Templates allow you to write a single piece of code that can work with different data types, which makes code more flexible and reusable.
Exception Handling: C++ has a built-in exception handling mechanism that allows you to write code that can handle unexpected errors or exceptions. This helps to make code more robust and reliable.
Standard Template Library (STL): C++ comes with a powerful library called the Standard Template Library (STL) that provides a wide range of useful data structures and algorithms. STL includes containers such as vectors, maps, and sets, and algorithms such as sorting and searching.
Pointers: Pointers are a powerful feature of C++ that allow you to work with memory directly. Pointers allow you to manipulate data at a low level and can be used to create more efficient code. However, they can also be a source of errors if not used carefully.
Virtual Functions: C++ allows you to define virtual functions, which are functions that can be overridden by derived classes. Virtual functions make it possible to write code that can be used with different types of objects, which makes code more flexible and reusable.
Multi-Threading: C++ provides a way to create multi-threaded applications, which can improve performance by allowing different parts of the program to execute concurrently. Multi-threading can be complex, but it is a powerful tool for creating high-performance software.
These are just a few examples of the many features and concepts in C++. C++ is a complex and powerful language that can be used to write a wide range of software, from low-level operating system code to high-level user interfaces. It is an important language to learn for anyone interested in software development.
Introduction to Object-Oriented Programming (OOP): In this topic, you’ll learn the basics of OOP, including the four fundamental concepts: encapsulation, inheritance, polymorphism, and abstraction. You’ll also learn about classes, objects, and methods.
C++ Basics: This topic covers the basic syntax and structure of C++ programs, including data types, variables, control structures, functions, and arrays.
Pointers and Memory Management: Pointers are one of the most powerful features of C++. They allow you to manipulate memory directly, which is essential for building efficient and flexible data structures. In this topic, you’ll learn how to use pointers, as well as techniques for managing memory in C++.
Classes and Objects: Classes are the heart of OOP, and in this topic, you’ll learn how to define and use classes in C++. You’ll also learn about constructors, destructors, access modifiers, and other features of C++ classes.
Inheritance and Polymorphism: Inheritance is a key concept in OOP, and C++ supports both single and multiple inheritance. In this topic, you’ll learn how to define and use inheritance relationships in C++, as well as how to use polymorphism to write more flexible and reusable code.
Templates and Standard Template Library (STL): Templates allow you to write generic code that can be used with different data types. The STL is a collection of reusable classes and functions that are designed to work with templates. In this topic, you’ll learn how to use templates and the STL to write more flexible and reusable code.
Exception Handling: Exception handling allows you to handle errors and unexpected events in your code. In this topic, you’ll learn how to use the C++ exception handling mechanism to catch and handle exceptions.
Input/Output (I/O) Streams: In C++, input and output operations are performed using streams. In this topic, you’ll learn how to use I/O streams to read and write data from files and other sources.
Data Structures and Algorithms: C++ is a powerful language for building data structures and algorithms. In this topic, you’ll learn about some of the most common data structures and algorithms, including arrays, linked lists, stacks, queues, trees, and sorting and searching algorithms.
Graphics and Multimedia: C++ is also used for developing graphics and multimedia applications. In this topic, you’ll learn about some of the popular graphics libraries and multimedia frameworks used in C++, such as OpenGL, SDL, and SFML.
These are the main topics that are covered in a typical C++ syllabus. Of course, the depth of coverage and specific topics may vary depending on the course or program. But with a solid understanding of these topics, you should have a strong foundation in C++ programming.
FAQ (frequently asked questions) about C++
A: C++ is a general-purpose programming language that was developed as an extension of the C programming language. It is an object-oriented language that supports data abstraction, encapsulation, inheritance, and polymorphism.
A: Some of the main features of C++ include: object-oriented programming, template classes, exception handling, operator overloading, and standard template library (STL).
A: C is a procedural programming language, while C++ is an object-oriented programming language. C++ supports classes, inheritance, encapsulation, and polymorphism, which are not present in C. C++ also has a richer set of features, such as templates, exception handling, and a standard template library (STL).
A: C++ is a versatile language that can be used for a wide range of applications, from operating systems and device drivers to game development and finance. Its performance and efficiency make it a popular choice for system-level programming, while its object-oriented features make it easy to write maintainable and reusable code.
A: C++ is used in a wide range of applications, including: operating systems (such as Windows and Linux), database management systems, game development, graphics and animation, financial analysis and modeling, and scientific computing.
A: Some common mistakes include: failing to properly manage memory (leading to memory leaks or crashes), not following good object-oriented design principles, overusing global variables, and relying too heavily on complex language features.
A: There are many resources available for learning C++, including: online tutorials and courses, textbooks, video lectures, forums and communities, and open-source projects. Some popular resources include: C++ Primer by Lippman, Lajoie, and Moo, the C++ FAQ, and the C++ Standard Library.