C Language Programming Class | C Language Course Details Syllabus | C C++ Training Academy Online
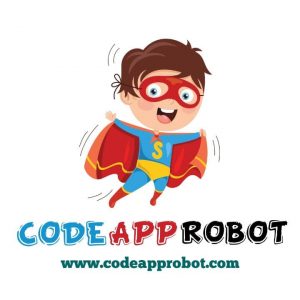
PRINT F , SCAN F
Write a program to print % d on the screen.
Write a program to print \n on the screen.
Write a program to print language on the screen.
Write a program to print hi in the first line and online in the second line.
Write a program to calculate area of a circle. Take radius as an input from user.
Write a program to calculate volume of a cuboid. Take user input .
Write a program to calculate area of a rectangle. Take length and breath from user.
Write a program to print “digital” on the screen.
Write a program to calculate simple interest. Take user input.
Write a program to calculate average of three numbers.
OPERATORS
Write a program to swap values of two int variables without using third variable.
Write a program to swap values of two int variables.
Write a program to print last digit of a given number.
Write a program to input a charater from keyboard and print its ASCII code.
Write a program to print a given number but without last digit.
DECISION CONTROL
Write a program to check whether a given number Is even or odd.
Write a program to check whether a given number is even or odd without using % operator.
Write a program to check whether a given number is positive or non positive.
Write a program to check whether a given number is positive, negative or zero.
Write a program to check whether a given number is divisible by 5 or not.
Write a program to check whether an year is a leap year or not.
Write a program to check nature of roots of a given quadratic equation.
Write a program which takes marks of 5 subjects ( assume maximum marks for each subject is 100 ) display result as pass or fail. Also print division obtained if candidate has passed the exam.
Write a program to find greater between two numbers.
Write a program to find greater among three numbers.
C Language Programming Class | C Language Course Details Syllabus | C C++ Training Academy Online
WHILE LOOP
Write a program to print first N Natural Numbers in Reverse order.
Write a program to print first 10 natural numbers.
Write a program to print first N Natural numbers.
Write a program to print first 10 natural numbers in Reverse order.
Write a program to print first 10 even natural numbers.
Write a program to print first 10 even natural numbers in Reverse order.
Write a program to print first N Even Natural numbers in Reverse order.
Write a program to print first N even natural numbers.
Write a program to print first 10 odd natural numbers in Reverse order.
Write a program to print first 10 odd natural numbers.
Write a program to print first N Odd natural numbers.
Write a program to print squares of first N Natural numbers.
Writ e a program to print first N odd natural numbers in Reverse order.
Write a program to calculate sum of first N Natuarl numbers.
LOOP
Write a program to calculate sum of first N odd natural numbers.
Write a program to calculate sum of squares of first N Natural numbers.
Write a program to calculate sum of cubes of first N Natural numbers.
Write a program to calculate factorial of a number.
Write a program to calculate sum of digits of a given number
Write a program to count digits in a given number.
Write a program to reverse a number.
Write a program to print first N terms of a Fibonacci series.
Write a program to print table of users choice.
Write a program to find Nth term of a Fibonacci series.
Write a program to check whether a given number is a term in Fibonacci series or not.
Write a program to print all prime numbers under 100.
Write a program to check whether a given number is prime or not,
Write a program to print next prime number of a given number.
Write a program to print all prime numbers between two given numbers.
Write a program to check whether two given numbers are co -prime or not.
Write a program to print all prime factors of a given number.
Write a program to print all factors of a given number.
Write a program to calculate HCF of two numbers.
Write a program to calculate LCM of two numbers.
Write a program to print first N prime numbers.
C Language Programming Class | C Language Course Details Syllabus | C C++ Training Academy Online
SWITCH
Write a menu driven program with following options
- Check whether a given set of three numbers are lengths of an isosceles triangle or not.
- Check whether a given set of three numbers are lengths of sides of a right angled triangle or not.
- Check whether a given set of three numbers are equilateral triangle or not.
Write a program which takes day number of a weel and display unique greeting message for the day.
Write a menu driven program with following options
- Addition
- Subtraction
- Multiplication
- Division
- Exit
Write a program which takes month number as an input and display number of days in that month.
FUNCTIONS
Write a function to calculate circumference of a circle ( TSRS )
Write a function to calculate area of circle ( TSRS )
Write a function to print first n even natural numbers ( TSRN )
Write a function to print first n natural numbers ( TSRN )
Write a function to calculate sum of squares of first n natural numbers ( TSRS )
Write a function to calculate sum of first n natural numbers ( TSRS )
Write a function to calculate factorial of a number ( TSRS )
Write a function to check whether a given number is even or odd ( TSRS ) ( Return 1 if even otherwise return 0 )
Write a function to print all Armstrong numbers in the given range.
Write a function to remove all occurrence of a given digit from a given number.
Write a function to check whether a given number is an Armstrong number or not.
Write a function to print all prime numbers between two given numbers ( TSRN )
Write a function to find next prime number of a given number ( TSRS )
Write a function to check whether a given number is prime or not ( TSRS ) ( Return 1 if prime , otherwise return 0 )
Write a function to calculate number of permutations which can be made from n items, selected r at a time ( TSRS ).
Write a function to calculate number of combinations which can be made from n items, selected r at a time ( TSRS ).
C Language Programming Class | C Language Course Details Syllabus | C C++ Training Academy Online
RECURSION
Write a recursive function to print first n natural numbers
Write a recursive function to print first n even natural numbers
Write a recursive function to print first n natural numbers in Reverse order.
Write a recursive function to print first n odd natural numbers.
Write a recursive function to print first n even natural numbers in reverse order.
Write a recursive function to calculate HCF of two numbers.
Write a recursive function to print reverse of a given number.
Write a recursive function to find nth term of a Fibonacci series.
Write a recursive function to print octal equivalent of a given decimal number.
Write a recursive function to calculate sum of first n odd natural numbers.
Write a recursive function to calculate sum of squares of first n natural numbers.
Write a recursive function to calculate sum of digits of a given number.
Write a recursive function to print binary equivalent of a given decimal number.
Write a recursive function to print first n odd natural numbers in reverse order.
Write a recursive function to calculate sum of first n natural numbers.
Write a recursive function to calculate sum of first n even natural numbers.
ARRAYS
Write a program to find the smallest element in an array of size 10.
Write a program to sort an array of size 10.
Write a program to find the greatest element in an array of size 10.
Write a program to calculate average of 10 numbers. Use arrays to store 10 numbers.
Write a program to calculate sum of 10 numbers stored in an array.
Write a Program to calculate sum of all even and sum of all odd numbers stored in an array of size 10.
ARRAYS AND FUNCTION
Write a function to find the index of the smallest element of an array of size n.
Write a function to print distinct elements of a given array.
Write a function to print frequency of each element of the array.
Write a function to rotate an array towards right by one position.
Write a function to reverse an array.
Write a function to sort an array of size n.
Write a function to print frequency of each element of the array.
Write a function to calculate mean deviation of given elements.
Write a function to calculate standard deviation of given elements.
2D ARRAYS
Write a program to calculate inverse of a 3 x 3 matix.
Write a program to calculate sum of two matrices each of order 3 x 3 .
Write a program to calculate product of two matrices each of order 3 x 3.
C Language Programming Class | C Language Course Details Syllabus | C C++ Training Academy Online
STRINGS
Write a program to convert a string into uppercase.
Write a program to calculate length of the string using strlen function.
Write a program to calculate length of the string without using strlen function.
Write a program to count occurrence of a given character in a given string.
Write a program to convert a string into lower case.
Write a program to reverse a string using strrev function.
Write a program to reverse a string without using strrev function.
STRINGS AND FUNCTIONS
Write a function to compare two strings
Write a function to capitalize a string
Write a function to count vowels in a given string.
Write a function to count words in a given string.
Write a function to reverse a string word wise.
Write a function to check whether a given string is alphanumeric or not .
Write a function to check whether a given string is palindrome or not.
Write a function to count occurrence of a given character in a given string.
Write a function to find index of first occurrence of a given character in a given string.
Write a function to perform case insensitive comparison between two strings.
Write a function to find a given pattern in a given string.
MULTIPLE STRINGS
Write a program to sort a set of 10 city names.
Write a program to count vowels in each of the string. When 8 strings are stored in a two dimensional char array. Also print the total number of vowels.
C Language Programming Class | C Language Course Details Syllabus | C C++ Training Academy Online
POINTER , STRUCTURE AND DMA
Define a structure book with bookid, title and price as member variables.
Define a structure to represent date.
Define a structure employee with empid, name and salary as member variables.
Write a function to swap two numbers.
Define a structure coordinate with two variables x and y. write a function which takes a coordinate as an argument and return quadrant number in which coordinate lies. Return 0 if coordinate lies on axes.
Write o program of linked list.
Write a function to sort an array of employees according to their salaries. ( use structure of quetion 3 )
Write a function to add two complex numbers. Define structure to handle a complex number.
Write a function to accept variable length string from keyboard.
Write a function which takes an array of integer values as an argument. Create two arrays ( use DMA ) and store all the non – negative values of given array in first newly created array and store all negative values in the second newly created array.